CSE2305
Object-Oriented Software Engineering
Exercise 3: Animal Hospital
This exercise is designed to reinforce the concepts discussed in the lectures.
There are no marks for completing this exercise, however your attendance at
tutorials and successful completion is officially recorded and may be used
as
a positive adjunct to assessment in special circumstances.
You are strongly advised to compete the exercise as it will help
you with the concepts discussed in lectures and assist you in successfully
completing
the practical work. Exercises often mysteriously reappear as exam
questions.
Topic: Create a simple database to help the animal
hospital
Purpose: a chance to gain experience with inheritance using
C++
Background: Cruella's hospital for lost animals looks after
a variety of animals while they are sick. Business has been growing in recent
months and Cruella has decided she needs to computerize to keep track of animals
and feeding schedules.
Your task is to develop a basic prototype system using C++ to help keep track
of animals kept in the hospital. For each animal, Cruella would like to keep
a record of the animal's name, age, sex, date arrived and estimated length of
stay. Different types of animals have different feeding schedules, for simplicity
let us assume this information is just the number of times per day each animal
needs to be fed.
We will also assume at this prototype stage we are only dealing with three
different classes of animal: dogs, cats, and worms (yes, worms). Here is a simple
class diagram:
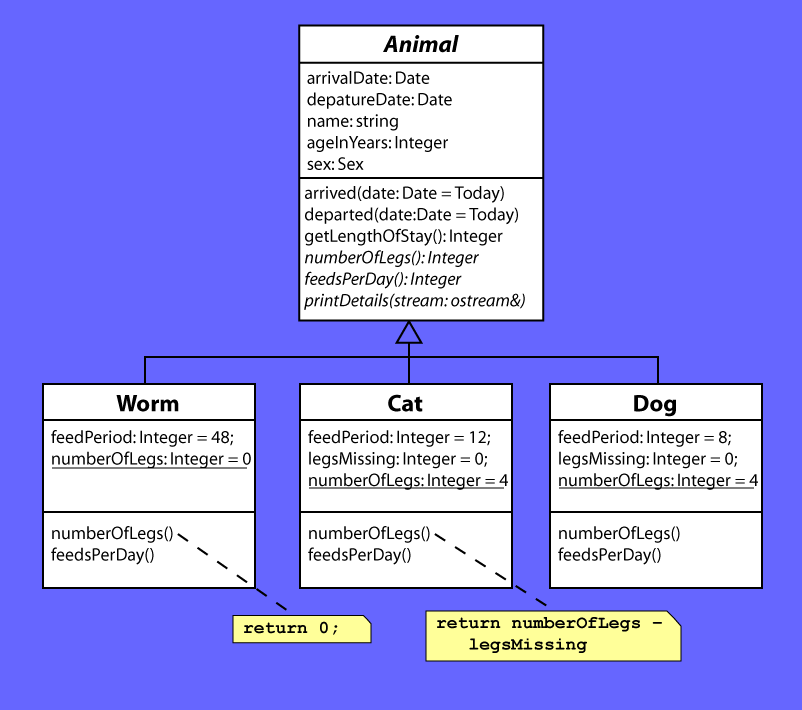
How to Proceed:
- Implement the class structure as shown in the diagram above.
- Make sure to keep interface and implementation in separate files (".c++"
and ".h").
- Also create an "AnimalHospital"
class that keeps an array of Animals,
can add, delete, and print the animals in the animal hospital.
- Test your AnimalHospital
class by creating a simple text interface to add an animal, delete an animal
and print animals in the hospital.
Hints/Questions:
- Use the Date class you created
from the previous exercise.
- Not all the interface information is provided in the class diagram above,
so you will have to add extra methods (e.g. constructors).
- Create new animals using the new operator, e.g.
Animal * a = new Cat("Sootie",
Female, 3);
Then add them to the AnimalHospital class:
cruellasHospital.add(a);
- Make sure you write a destructor for AnimalHospital
– when you add a new animal the responsibility for the animal transfers
to AnimalHospital. What happens
if some other class also is referencing the object held by AnimalHospital?
- There might seem to be a lot of similar code between Dog
and Cat – could
the hierarchy in the diagram above be changed by adding a new class that deals
with the common properties of Dog
and Cat? Is this a better structure?
Why?
- Could the class attributes (e.g. feedPeriod)
be shifted to the base class based on commonality?
This material is part of the CSE2305 – Object-Oriented
Software Engineering course.
Copyright © Jon McCormack, 2005. All rights reserved.
Last modified:
August 11, 2005